选择window验证登陆方式
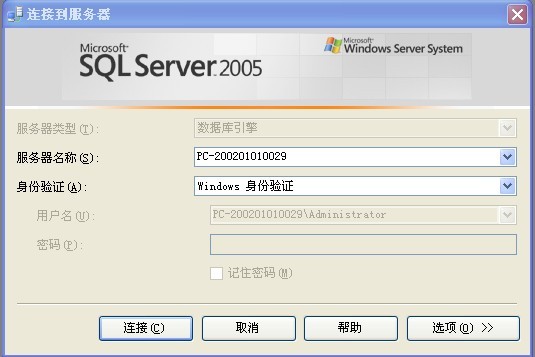
整个程序如下:用户登录--进入用户文章页面--可对文章进行增删改
首先打开VS2010 文件--新建--网站
确定
接着是数据库,这里用SQL2005
选择window验证登陆方式
进入数据库,点新建查询,建库加建表
create database Test use Test create table UserInfo ( id int primary key identity(1,1), username varchar(20), password varchar(20) ) create table Article ( id int primary key identity(1,1), title varchar(50), content varchar(100), uid int FOREIGN KEY REFERENCES UserInfo(id) ) insert into UserInfo values('test','123')
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Data; using System.Data.SqlClient; /// <summary> ///DBcon 的摘要说明 /// </summary> public class DBcon { private static SqlConnection sqlcon; public DBcon() { // //TODO: 在此处添加构造函数逻辑 // } public static SqlConnection getconnection() { sqlcon = new SqlConnection("Data Source=.;Integrated Security=SSPI;Initial Catalog=Test"); return sqlcon; } }
<html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> </head> <body> <form id="form1" runat="server"> <div> <table> <tr><td>用户名:</td><td><asp:TextBox ID="txtusername" runat="server"></asp:TextBox></td></tr> <tr><td>密码:</td><td><asp:TextBox ID="txtpassword" runat="server" TextMode="Password"></asp:TextBox></td></tr> <tr><td colspan="2"> <asp:Button ID="btnLogin" runat="server" Text="登录" /></td></tr> </table> </div> </form> </body> </html>
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data; using System.Data.SqlClient; public partial class Login : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void btnLogin_Click(object sender, EventArgs e) { if(this.txtusername.Text.Trim()=="" || this.txtpassword.Text.Trim()=="") Response.Write("<script>alert('用户名或密码不能为空')</script>"); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Data; using System.Data.SqlClient; /// <summary> ///Method 的摘要说明 /// </summary> public class Method { public Method() { // //TODO: 在此处添加构造函数逻辑 // } /// <summary> /// 对数据插入,更改,删除方法 /// </summary> /// <param name="sql">SQL语句</param> public static void DataMethod(string sql) { SqlConnection mycon = DBcon.getconnection(); mycon.Open(); SqlCommand cmd = new SqlCommand(sql, mycon); try { cmd.ExecuteNonQuery(); } catch (Exception ex) { throw (ex); } finally { mycon.Dispose(); mycon.Close(); } } /// <summary> /// 检查数据 /// </summary> /// <param name="sql"></param> /// <returns></returns> public static bool DataCheak(string sql) { SqlConnection mycon = DBcon.getconnection(); mycon.Open(); SqlCommand cmd = new SqlCommand(sql, mycon); SqlDataReader dr = cmd.ExecuteReader(); bool b = false; if (dr.Read()) { b = true; } dr.Dispose(); cmd.Dispose(); mycon.Dispose(); return b; } /// <summary> /// 以DataSet获取数据 /// </summary> /// <param name="sql"></param> /// <param name="table"></param> /// <returns></returns> public static DataSet GetData(string sql, string table) { SqlConnection mycon = DBcon.getconnection(); SqlCommand cmd = new SqlCommand(sql, mycon); try { SqlDataAdapter da = new SqlDataAdapter(cmd); DataSet ds = new DataSet(); da.Fill(ds, table); return ds; } catch (Exception ex) { throw (ex); } finally { mycon.Dispose(); mycon.Close(); } } public static SqlDataReader GetData(string sql) { SqlConnection mycon = DBcon.getconnection(); mycon.Open(); SqlCommand cmd = new SqlCommand(sql, mycon); try { SqlDataReader dr = cmd.ExecuteReader(CommandBehavior.CloseConnection); return dr; } catch (Exception ex) { throw (ex); } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Data; using System.Data.SqlClient; /// <summary> ///TestService 的摘要说明 /// </summary> public class TestService { public TestService() { // //TODO: 在此处添加构造函数逻辑 // } public Boolean Login(string username,string password) { String sql = "select * from UserInfo where username='"+username+"' and password='"+password+"'"; if (Method.DataCheak(sql)) return true; else return false; } public DataSet ShowList(int Userid) { String sql = "select * from Article where uid="+Userid+""; DataSet ds = Method.GetData(sql, "list"); return ds; } public DataSet GetUser(string username, string password) { String sql = "select * from UserInfo where username='"+username+"' and password='"+password+"'"; DataSet ds = Method.GetData(sql, "UserInfo"); return ds; } public void Add(string title, string content,int uid) { String sql = string.Format("insert into Article values('{0}','{1}',{2})", title, content, uid); Method.DataMethod(sql); } public SqlDataReader GetOneList(int id) { String sql = "select * from Article where id="+id+""; SqlDataReader dr = Method.GetData(sql); return dr; } public void Update(string title, string content, int id) { String sql = "update Article set title='" + title + "',content='" + content + "' where id=" + id + " "; Method.DataMethod(sql); } public void Delete(int id) { String sql = "delete from Article where id=" + id + " "; Method.DataMethod(sql); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data; using System.Data.SqlClient; public partial class Login : System.Web.UI.Page { TestService ts = new TestService(); protected void Page_Load(object sender, EventArgs e) { } protected void btnLogin_Click(object sender, EventArgs e) { if(this.txtusername.Text.Trim()=="" || this.txtpassword.Text.Trim()=="") Response.Write("<script>alert('用户名或密码不能为空')</script>"); else if (!ts.Login(this.txtusername.Text.Trim(), this.txtpassword.Text.Trim())) Response.Write("<script>alert('用户名或密码错误')</script>"); else { DataSet ds = ts.GetUser(this.txtusername.Text.Trim(), this.txtpassword.Text.Trim()); Session["UID"] = Convert.ToInt32(ds.Tables["UserInfo"].Rows[0][0].ToString()); Session["Username"] = ds.Tables["UserInfo"].Rows[0][1].ToString(); Response.Write("<script>alert('登录成功');location.href='ArticleList.aspx'</script>"); } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data; using System.Data.SqlClient; public partial class ArticleList : System.Web.UI.Page { TestService ts = new TestService(); protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { this.Label1.Text = Session["Username"].ToString(); DLBind(); } } public void DLBind() { DataSet ds = ts.ShowList(int.Parse(Session["UID"].ToString())); this.Dl_List.DataSource = ds.Tables["List"].DefaultView; this.Dl_List.DataBind(); if (ds.Tables[0].Rows.Count <= 0) { sorry.Style["display"] = "block"; } } protected void LB_Add_Click(object sender, EventArgs e) { Response.Redirect("Add.aspx"); } protected void Dl_List_ItemCommand(object source, DataListCommandEventArgs e) { switch (e.CommandName) { case "delete": ts.Delete(int.Parse(Dl_List.DataKeys[e.Item.ItemIndex].ToString())); Response.Write("<script>alert('删除成功')</script>"); DLBind(); break; } } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class Add : System.Web.UI.Page { TestService ts = new TestService(); protected void Page_Load(object sender, EventArgs e) { } protected void btnAdd_Click(object sender, EventArgs e) { string title=this.txtTitle.Text.Trim(); string content=this.txtContent.Text.Trim(); int uid=int.Parse(Session["UID"].ToString()); ts.Add(title, content, uid); Response.Write("<script>alert('添加成功');location.href='ArticleList.aspx'</script>"); } }
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; using System.Data; using System.Data.SqlClient; public partial class Update : System.Web.UI.Page { TestService ts = new TestService(); protected void Page_Load(object sender, EventArgs e) { if (!IsPostBack) { ListBind(Convert.ToInt32(Request.QueryString["id"].ToString())); } } public void ListBind(int id) { SqlDataReader dr = ts.GetOneList(id); if (dr.Read()) { this.txtTitle.Text = dr["title"].ToString(); this.txtContent.Text = dr["content"].ToString(); } } protected void btnUpdate_Click(object sender, EventArgs e) { ts.Update(this.txtTitle.Text, this.txtContent.Text, Convert.ToInt32(Request.QueryString["id"].ToString())); Response.Write("<script>alert('修改成功');location.href='ArticleList.aspx'</script>"); } }